webpack 基础(三): 核心概念
Entry
entry会根据入口文件分析依赖生成依赖关系图。
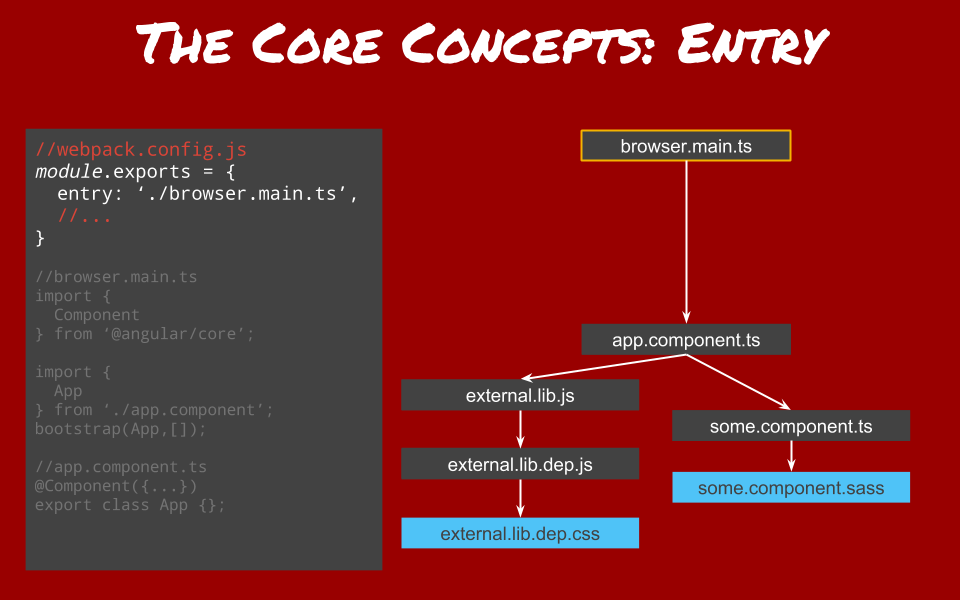
Ouput
告诉 Webpack 如何及在哪里分发 bundles (编译后文件),和 Entry 一起工作。
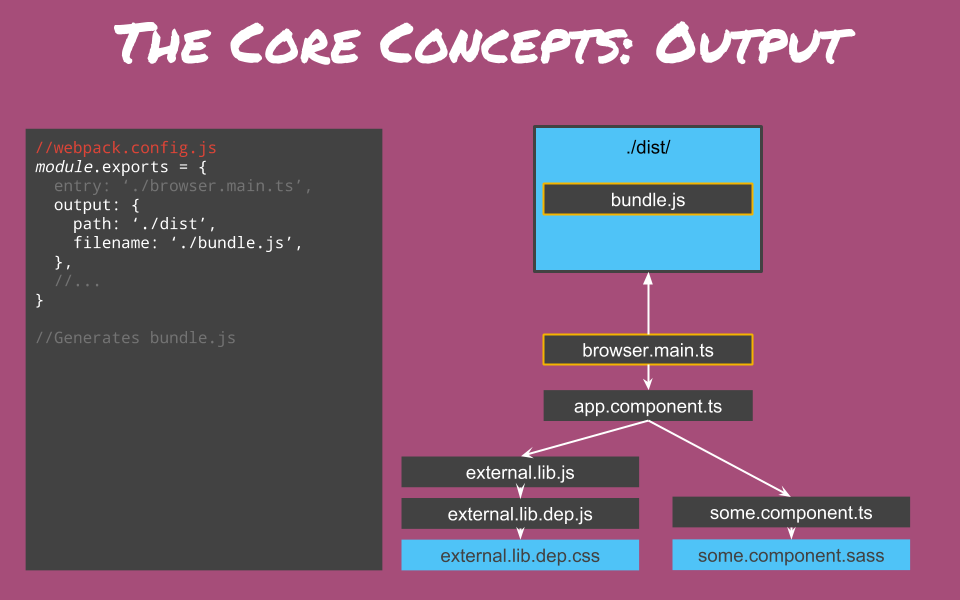
Loader+Rules
loaders
Webpack 4.0 中将每一个依赖都当作一个JavaScript Module处理。所以我们需要定义一个规则,如何把一个文件当作JavaScript文件处理。Webpack处理文件依赖的时候,遇到这些文件就会去rules中寻找相对应的loader,处理后将文件加入依赖关系图。
1 | module: { |
test: 一个正则表达式,指定compiler运行loader的文件格式
use: 使用loader对象的array/string/function
enforce: 可以是"pre"or"post",告诉Webpack这条规则在所有其他规则前面或者后面。
include: 一个正则表达式数组,用来指示compiler哪些文件或者文件夹被包含。complier将仅搜索提供的路径。
exclude: 一个正则表达式数组,用来指示compiler哪些文件或者文件夹被忽略。
(链式loaders)chaining loaders
1 | rules: [ |
假设我们有一个style.less文件,那么它的调用顺序就是 style.less => less-loader => css-loader => style-loader => inlineStyleBrowser.js。
当然Webpack能通过loader处理大量的文件,如下所示:
1 | son, hson, raw, val, to-string, imports, exports, expose, script, apply, callback, ifdef-loader, source-map, sourceMappingURL, |
Plugins
首先Plugin是一个带有apply属性的对象,它能允许挂载到整个编译生命周期。Webpack本身拥有丰富的内置插件。
首先来看一个插件例子:
1 | // webpack 3.0 api |
我们需要做的就是 new 一个新的实例放到plugins里面。这样有一些基础的插件也能够被复用和修改。
下面是调用的例子:
1 | var BellOnBundlerErrorPlugin = require(‘bell-on-error’); |
Webpack的80%都是由它自己的插件系统构成的。相较于loader知识处理文件转换成模块,plugin能做的更多。它能访问ComplierAPI,在编译期间做一些事情。
总结
Entry: 告诉 Webpack 哪些文件需要加载到浏览器;编译输出到 output 属性中。
Output: 告诉 Webpack 如何及在哪里分发 bundles (编译后文件),和 Entry 一起工作。
Loaders: 告诉Webpack如何解释和翻译文件。再将文件添加到依赖关系图之前,对每个文件进行转换。
Plugins: 为编译过程添加更多的功能模块。又有更强大的访问ComplierAPI的能力。
- 本文标题:webpack 基础(三): 核心概念
- 本文作者:hddhyq
- 本文链接:https://hddhyq.github.io/2019/04/07/Webpack基础3/
- 版权声明:本博客所有文章除特别声明外,均采用 CC BY-NC-SA 4.0 许可协议。转载请注明出处!